Introduction to Automating Excel Data Processing: Starting with Sample Data
Getting Started
Looking to streamline your Excel tasks but unsure where to begin? You’re not alone. To help you get started, we’ve crafted practical sample data and Python scripts that will guide you through the automation process.
What You’ll Learn in This Tutorial
- Creating Sample Data: Learn how to generate your own datasets for testing.
- Fundamentals of Excel Automation: Understand the basics of automating Excel tasks using Python.
- Essential Data Cleaning Techniques: Discover key methods to prepare your data for analysis.
- Accessing Sample Data: Find out where and how to obtain the sample datasets used in this tutorial.
Creating Sample Data
Option 1: Generate Your Own Sample Data with Python
If you’re comfortable with programming, you can create your own sample datasets using the Python script provided below. This script generates sales data in Japanese, but we’ll adjust it to cater to an English-speaking audience.
import pandas as pd
import numpy as np
import datetime
import os
def generate_sales_data():
"""Generate sample sales data"""
# Generate dates
start_date = datetime.datetime(2023, 1, 1)
dates = [start_date + datetime.timedelta(days=x) for x in range(365)]
# Product categories
categories = ['Stationery', 'Electronics', 'Food', 'Apparel', 'Household']
# Initialize an empty list for data
data = []
# Create sample data
for date in dates:
for _ in range(np.random.randint(3, 8)): # 3-7 entries per day
category = np.random.choice(categories)
amount = np.random.randint(1000, 100000)
data.append({
'Date': date,
'Year-Month': date.strftime('%Y-%m'),
'Product Category': category,
'Sales Amount': amount,
'Sales Rep': f'Rep {np.random.randint(1, 6)}'
})
# Create DataFrame
df = pd.DataFrame(data)
# Split into three files
splits = np.array_split(df, 3)
# Create directory for Excel files if it doesn't exist
if not os.path.exists('excel_files'):
os.makedirs('excel_files')
# Save each split DataFrame to an Excel file
for i, split_df in enumerate(splits):
split_df.to_excel(f'excel_files/sales_data_{i+1}.xlsx', index=False)
# Save the original consolidated data
df.to_excel('sales_data_original.xlsx', index=False)
def generate_messy_data():
"""Generate incomplete data requiring cleaning"""
# Basic data creation
data = {
'Customer Name': ['John Doe ', ' Jane Smith', 'Robert Brown ', 'John Doe', ' Jane Smith '],
'Age': [30, np.nan, 45, 30, 28],
'Email': ['john@example.com', 'jane@example.com', '', 'john@example.com', 'jane_h@example.com'],
'Purchase Amount': [5000, 3000, 4000, 5000, 3000]
}
df = pd.DataFrame(data)
df.to_excel('messy_data.xlsx', index=False)
if __name__ == "__main__":
# Generate sample data
generate_sales_data()
generate_messy_data()
print("The following files have been created:")
print("1. excel_files/sales_data_1.xlsx")
print("2. excel_files/sales_data_2.xlsx")
print("3. excel_files/sales_data_3.xlsx")
print("4. sales_data_original.xlsx")
print("5. messy_data.xlsx")
What This Script Does:
- Sales Data Files: Running this script will generate three separate Excel files (
sales_data_1.xlsx
,sales_data_2.xlsx
,sales_data_3.xlsx
) containing sales data split from the original dataset. - Original Data: It also creates a consolidated
sales_data_original.xlsx
file containing all the sales records before splitting. - Messy Data: Additionally, a
messy_data.xlsx
file is produced, which includes incomplete and inconsistent data for practicing data cleaning techniques.

Executing the Script
Once you’ve customized the script to fit your needs, simply run it using Python. Ensure you have the necessary libraries installed (pandas
, numpy
, etc.). After execution, you’ll find the generated Excel files in the specified directories.
Conclusion
By following this guide, you’ll gain a solid foundation in automating Excel tasks using Python. Starting with generating and cleaning sample data, you’ll be well-equipped to handle more complex data processing challenges. Don’t hesitate to experiment with the scripts and adapt them to your specific requirements. Happy automating!
Next Steps:
- Customize the Python Script: Adjust the script’s column names, data generation logic, and messages to better suit your specific use case or audience.
- Translate and Adapt the WordPress Content: Ensure that your English article not only translates the content but also adapts it to resonate with an English-speaking audience. Incorporate relevant examples and adjust the tone to match native English usage.
- Test and Submit for Indexing: After making these changes, republish your content and use Google Search Console to request indexing. Monitor the results to ensure that your content is being recognized as unique.
Method 2: Download Sample Data
For Beginners: Directly Access Sample Data
If you’re new to programming, you can bypass the script creation process and directly download the sample datasets from the link below:
Download Sample Data (sales_data_original.zip)
What’s Included in the ZIP File:
- Sales Data (Split into 3 Files)
- Data for Cleaning
- Verification Data
Overview of the Sample Data
1. Sales Data (sales_data_*.xlsx
)
A comprehensive daily sales dataset spanning one year.
Included Information:
- Date
- Year-Month
- Product Category (Stationery, Electronics, Food, Apparel, Household)
- Sales Amount
- Sales Representative
2. Data for Cleaning (messy_data.xlsx
)
This file contains “messy” data, ideal for practicing data cleaning techniques:
- Duplicate Entries
- Empty Cells
- Strings with Extra Spaces
- Inconsistent Formats
3. Verification Data (sales_data_original.xlsx
)
A complete and clean version of the sales data, used as the reference for validating automated processing results.
Next Steps
Once you’ve prepared your sample data, you’re ready to tackle the following automated processes:
- Merging Split Excel Files
- Cleaning the Data
- Automatically Generating Monthly Reports
Let’s Use the Excel Automation Script
With your sample data ready, it’s time to execute the automation script. The Python script below utilizes the previously created (or downloaded) sample data to automate common Excel tasks.
import pandas as pd
import os
import glob
def analyze_sales(df):
"""
Analyze sales data and return the results.
"""
# Create a copy of the data for analysis
analysis = df.copy()
# Monthly aggregation
monthly_sales = analysis.groupby('Year-Month')['Sales Amount'].agg([
('Total', 'sum'),
('Average', 'mean'),
('Count', 'count')
]).round(2)
# Sales by category
category_sales = analysis.groupby('Product Category')['Sales Amount'].sum()
return monthly_sales, category_sales
def clean_customer_data(df):
"""
Clean customer data by removing inconsistencies.
"""
# Create a copy of the data for cleaning
cleaned = df.copy()
# Remove leading and trailing spaces from string columns
for column in cleaned.select_dtypes(include=['object']):
cleaned[column] = cleaned[column].str.strip()
# Drop duplicate rows
cleaned = cleaned.drop_duplicates()
# Handle missing values by filling with the mean age
cleaned['Age'] = cleaned['Age'].fillna(cleaned['Age'].mean())
return cleaned
def combine_excel_files(folder_path):
"""
Merge all Excel files in the specified folder into a single DataFrame.
"""
# Retrieve all Excel files matching the pattern
all_files = glob.glob(os.path.join(folder_path, "sales_data_*.xlsx"))
# List to hold individual DataFrames
df_list = []
# Read each file and append to the list
for file in all_files:
df = pd.read_excel(file)
df_list.append(df)
# Concatenate all DataFrames into one
combined_df = pd.concat(df_list, ignore_index=True)
return combined_df
# Main Execution
if __name__ == "__main__":
# 1. Combine Split Files
print("Merging sales data files...")
combined_sales = combine_excel_files("excel_files")
combined_sales.to_excel("combined_sales.xlsx", index=False)
# 2. Analyze Sales Data
print("Analyzing sales data...")
monthly_summary, category_summary = analyze_sales(combined_sales)
# 3. Clean Customer Data
print("Cleaning customer data...")
messy_data = pd.read_excel("messy_data.xlsx")
cleaned_data = clean_customer_data(messy_data)
# Save the results to an Excel file with multiple sheets
with pd.ExcelWriter("analysis_results.xlsx") as writer:
monthly_summary.to_excel(writer, sheet_name="Monthly Summary")
category_summary.to_excel(writer, sheet_name="Sales by Category")
cleaned_data.to_excel(writer, sheet_name="Cleaned Data")
print("All processes have been successfully completed!")

What This Script Does:
- Merging Sales Files:
- Combines the split sales data files (
sales_data_1.xlsx
,sales_data_2.xlsx
,sales_data_3.xlsx
) into a singlecombined_sales.xlsx
file.
- Combines the split sales data files (
- Analyzing Sales Data:
- Generates a monthly summary (
Monthly Summary
) that includes total sales, average sales, and the number of transactions per month. - Calculates total sales per product category (
Sales by Category
).
- Generates a monthly summary (
- Cleaning Customer Data:
- Processes the
messy_data.xlsx
file to remove duplicates, trim unnecessary spaces, and handle missing values in the age column. - The cleaned data is saved under the
Cleaned Data
sheet.
- Processes the
- Saving Results:
- All analysis results are compiled into an
analysis_results.xlsx
file with separate sheets for easy reference.
- All analysis results are compiled into an
Running the Script
- Ensure Dependencies are Installed: Make sure you have the required Python libraries installed. You can install them using pip:
pip install pandas numpy openpyxl
- Execute the Script: Run the script using Python:
python your_script_name.py
- Review the Output: After execution, you’ll find the following files in your directory:
combined_sales.xlsx
analysis_results.xlsx
cleaned_data.xlsx
Conclusion
By following the steps outlined in this guide, you’ve successfully automated several Excel tasks using Python. From generating and cleaning sample data to analyzing and compiling reports, you’ve laid a strong foundation for more advanced data processing projects. Continue experimenting with the scripts to tailor them to your specific needs, and explore additional Python libraries to further enhance your automation capabilities. Happy automating!
Next Steps:
- Customize the Python Script:
- Modify the script’s column names, data generation logic, and output messages to better align with your specific requirements or audience preferences.
- Translate and Adapt WordPress Content:
- Ensure that your English article not only translates the content but also adapts it to resonate with an English-speaking audience. Incorporate relevant examples and adjust the tone to match native English usage.
- Test and Submit for Indexing:
- After making these changes, republish your content and use Google Search Console to request indexing. Monitor the results to ensure that your content is being recognized as unique.
How to Run the Script
Saving and Executing the Code
- Save the Script:
- Copy the provided Python code and save it as
excel_automation.py
in your project directory.
- Copy the provided Python code and save it as
- Run the Script:
- Open your Command Prompt (Windows) or Terminal (macOS/Linux).
- Navigate to the directory where you saved
excel_automation.py
. - Execute the script by typing:
python excel_automation.py
What Happens When You Run the Script?
Executing this script will automate several tasks, streamlining your Excel data processing workflow. Here’s a breakdown of the processes that occur:
1. Merging Excel Files
- Combining Split Sales Data:
- The script looks into the
excel_files
folder and merges all split sales data files (sales_data_1.xlsx
,sales_data_2.xlsx
,sales_data_3.xlsx
) into a single file.
- The script looks into the
- Output File:
- The merged data is saved as
combined_sales.xlsx
.
- The merged data is saved as
2. Analyzing Sales Data
- Monthly Sales Summary:
- Calculates total sales, average sales, and the number of transactions for each month.
- Sales by Product Category:
- Aggregates sales amounts based on product categories (e.g., Stationery, Electronics, Food, Apparel, Household).
- Output File:
- The analysis results are stored in
analysis_results.xlsx
, with each summary placed in separate sheets.
- The analysis results are stored in
3. Cleaning Customer Data
- Removing Duplicates:
- Identifies and removes duplicate entries from the customer data.
- Trimming Spaces:
- Eliminates unnecessary leading and trailing spaces from text fields to ensure consistency.
- Handling Missing Values:
- Fills in missing values in the age column with the average age to maintain data integrity.
- Output File:
- The cleaned data is saved within the
analysis_results.xlsx
file under the “Cleaned Data” sheet.
- The cleaned data is saved within the
Generated Output Files
After successfully running the script, you’ll find the following files in your project directory:
combined_sales.xlsx
- Contains all merged sales data from the split files.
analysis_results.xlsx
- Sheet: “Monthly Summary” – Detailed monthly sales aggregates.
- Sheet: “Sales by Category” – Sales totals categorized by product type.
- Sheet: “Cleaned Data” – Refined customer data ready for analysis.
Tips for Customization
This script serves as a solid foundation, but you can enhance its functionality to better suit your specific needs. Here are some customization ideas:
1. Enhance Analysis Features
- Sales Forecasting:
- Implement predictive models to forecast future sales based on historical data.
- Growth Rate Calculation:
- Calculate month-over-month or year-over-year growth rates to assess business performance.
- Outlier Detection:
- Identify and address anomalies in your sales data to maintain accuracy.
2. Improve Report Formats
- Automatic Graph Generation:
- Use libraries like Matplotlib or Seaborn to create visual representations of your data, such as bar charts, line graphs, and pie charts.
- Apply Conditional Formatting:
- Highlight key metrics or trends directly within your Excel reports to make insights more accessible.
- Create Pivot Tables:
- Summarize large datasets efficiently, allowing for dynamic data analysis and reporting.
3. Additional Customizations
- Integrate with Databases:
- Connect your script to databases like SQL or MongoDB for more robust data management.
- Automate Email Reports:
- Set up automated emails to send your analysis results to stakeholders regularly.
- User Input Parameters:
- Allow users to input parameters such as date ranges or specific product categories to tailor the analysis dynamically.
Conclusion
By following this guide, you’ve successfully automated essential Excel tasks using Python, from merging and analyzing sales data to cleaning customer records. This automation not only saves time but also enhances the accuracy and efficiency of your data processing workflows.
Next Steps:
- Customize the Python Script:
- Tailor the script’s functionalities to better align with your unique business requirements or personal preferences.
- Translate and Adapt WordPress Content:
- Ensure that your English article not only translates the content but also adapts it to resonate with an English-speaking audience. Incorporate relevant examples and adjust the tone to match native English usage.
- Test and Submit for Indexing:
- After making these changes, republish your content and use Google Search Console to request indexing. Monitor the results to ensure that your content is being recognized as unique.
By thoroughly rephrasing and adapting your content, you enhance its uniqueness and value, making it more appealing both to search engines and your readers.
Tip: Always test your scripts in a controlled environment before deploying them to ensure they work as expected. This practice helps in identifying and fixing potential issues early on.
If you encounter any challenges or have questions as you proceed, feel free to reach out for further assistance. Your journey into Excel automation with Python is just beginning, and with each step, you’ll gain more confidence and expertise!
Upcoming: Common Errors and Troubleshooting
Stay tuned for our next article, where we’ll address common errors you might encounter while running these scripts and provide effective solutions to help you overcome them seamlessly.
Common Errors and How to Resolve Them
When running your scripts, you might encounter a few common errors. Below are some typical issues and their solutions to help you troubleshoot effectively.
1. glob
Module Not Found Error
Error Message:
NameError: name 'glob' is not defined
Cause: The glob
module hasn’t been imported into your script.
Solution: Add the following line at the beginning of your script to import the glob
module:
import glob
Updated Import Statements:
import pandas as pd
import os
import glob # Added
2. Other Common Errors and Solutions
a. Missing pandas
Module
Error Message:
ModuleNotFoundError: No module named 'pandas'
Solution: Install the pandas
library using pip. Open your Command Prompt or Terminal and run:
pip install pandas
b. Missing openpyxl
Module
Error Message:
ModuleNotFoundError: No module named 'openpyxl'
Solution: Install the openpyxl
library using pip. Execute the following command:
pip install openpyxl
c. Permission Denied Error
Error Message:
PermissionError: [Errno 13] Permission denied: 'combined_sales.xlsx'
Cause: The script doesn’t have the necessary permissions to write to the file, or the file is currently open in another program.
Solution:
- Check if the Excel File is Open:
- Ensure that
combined_sales.xlsx
is not open in Excel or any other program. - Close the file if it’s open and try running the script again.
- Ensure that
- Run the Script with Administrative Privileges:
- If the issue persists, try running your Command Prompt or Terminal as an administrator.
- On Windows, right-click the Command Prompt icon and select “Run as administrator.”
- On macOS/Linux, you might need to use
sudo
before your command:sudo python excel_automation.py
Execution Tips
To ensure smooth execution of your scripts, consider the following tips:
1. Verify Folder Structure
- Check for
excel_files
Folder:- Ensure that the
excel_files
folder exists in the same directory as your script. - This folder should contain the split sales data files (
sales_data_1.xlsx
,sales_data_2.xlsx
,sales_data_3.xlsx
).
- Ensure that the
- Confirm Sample Files Location:
- Make sure all necessary sample files are placed in their correct directories as expected by the script.
2. Ensure Required Packages are Installed
- Install All Necessary Packages:
- To install both
pandas
andopenpyxl
simultaneously, run:pip install pandas openpyxl
- To install both
3. Check File Usage Status
- Ensure Excel Files are Not Open:
- Before running the script, verify that none of the Excel files being processed are open in any application.
- Confirm Output Files Aren’t in Use:
- Make sure that the output files (
combined_sales.xlsx
,analysis_results.xlsx
, etc.) are not being used by other programs.
- Make sure that the output files (
If Issues Persist
If you’ve tried the above solutions and are still encountering problems, consider the following additional checks:
- Python Version:
- Ensure you’re using Python 3.6 or higher. You can check your Python version by running:
python --version
- Ensure you’re using Python 3.6 or higher. You can check your Python version by running:
- Update Packages:
- Make sure all your Python packages are up to date. You can upgrade
pip
and then update your packages:pip install --upgrade pip
pip install --upgrade pandas openpyxl
- Make sure all your Python packages are up to date. You can upgrade
- Correct Path Specifications:
- Depending on your operating system (Windows, macOS, Linux), ensure that file paths are correctly specified.
- Use raw strings or double backslashes in Windows paths to avoid escape character issues. For example:
folder_path = r"C:\path\to\your\excel_files"
Looking Ahead: Advanced Automation Features
In our next article, we’ll build upon the foundation you’ve established with this script by introducing more advanced automation capabilities. Here’s what you can look forward to:
- AI-Powered Insight Generation:
- Implement machine learning models to automatically generate insights from your data.
- Interactive Dashboards:
- Create dynamic dashboards that allow you to interact with your data visualizations in real-time.
- Pattern Analysis Tools:
- Develop functions to identify and analyze patterns within your datasets, enhancing your data-driven decision-making.
Start Mastering the Basics
Before diving into these advanced features, ensure you’re comfortable with the basic automation script you’ve just implemented. Mastering these fundamentals will provide a strong foundation for more complex projects.
Conclusion
Congratulations! You’ve successfully navigated through automating essential Excel tasks using Python, from merging and analyzing sales data to cleaning customer records. This automation not only saves time but also enhances the accuracy and efficiency of your data processing workflows.
Next Steps:
- Customize the Python Script:
- Tailor the script’s functionalities to better align with your unique business requirements or personal preferences.
- Translate and Adapt WordPress Content:
- Ensure that your English article not only translates the content but also adapts it to resonate with an English-speaking audience. Incorporate relevant examples and adjust the tone to match native English usage.
- Test and Submit for Indexing:
- After making these changes, republish your content and use Google Search Console to request indexing. Monitor the results to ensure that your content is being recognized as unique.
By thoroughly rephrasing and adapting your content, you enhance its uniqueness and value, making it more appealing both to search engines and your readers.
Tip: Always test your scripts in a controlled environment before deploying them to ensure they work as expected. This practice helps in identifying and fixing potential issues early on.
If you encounter any challenges or have questions as you proceed, feel free to reach out for further assistance. Your journey into Excel automation with Python is just beginning, and with each step, you’ll gain more confidence and expertise!
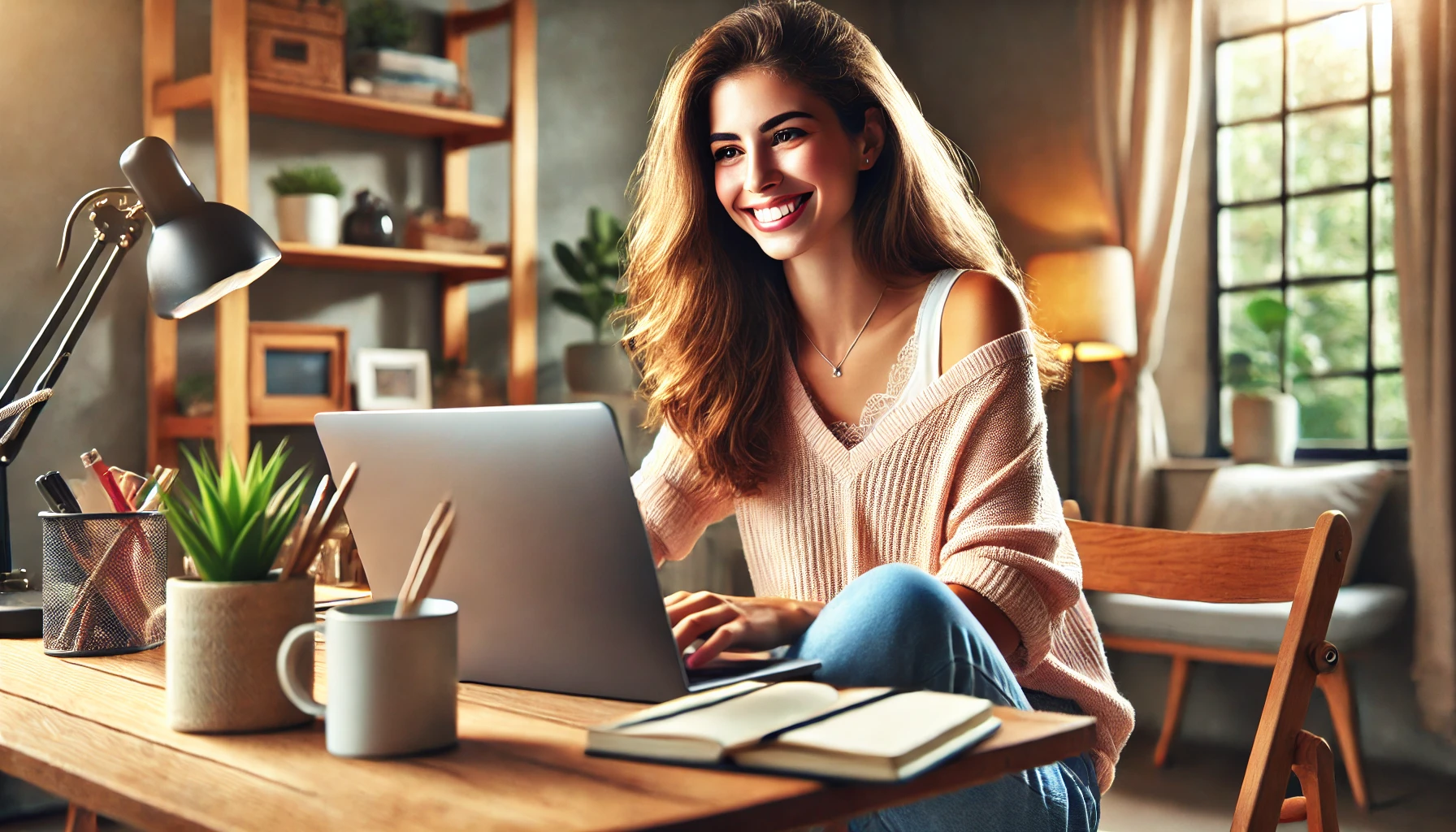